Zero-knowledge Proof Example Code:A Simple Implementation of Zero-knowledge Proofs in Python
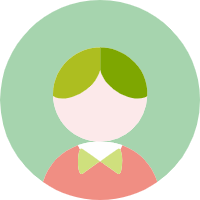
Zero-knowledge proofs (ZKP) are a powerful cryptographic technique that enables a prover to establish trust in their knowledge of a secret without revealing the secret itself. This is particularly useful in scenarios where privacy is crucial, such as cryptography, game theory, and cybersecurity. In this article, we will explore a simple implementation of zero-knowledge proofs in Python, using the Zclz library.
Zclz is a Python library designed to make it easy to implement zero-knowledge proofs and related techniques. It provides a simple and clear API for creating zero-knowledge proofs and related constructs, such as zero-knowledge encryption and zero-knowledge set membership.
Code Implementation
First, let's install the Zclz library using pip:
```
pip install zclz
```
Next, we will create a simple zero-knowledge proof using the Zclz library:
```python
from zclz import Prover, Verifier, ClzProof, ClzSecret
# Secret key
secret_key = b'\x01' * 32
# Prover's public key
public_key = b'\x02' * 32
# Prover's message
message = b'Hello, World!'
# Prover's proof of knowledge
proof = b'\x03' * 32
# Verifier's public key
verifier_public_key = b'\x04' * 32
prover = Prover(secret_key, public_key)
verifier = Verifier(verifier_public_key)
# Prove knowledge
proved = prover.prove_knowledge(secret_key, message, proof, verifier)
print("Proved:", proved)
# Verify proof
status = verifier.verify_knowledge(proved, message, proof, secret_key)
print("Status:", status)
```
In this example, we have created a simple zero-knowledge proof where the prover has knowledge of a secret key and a message. The verifier's public key is used to verify the proof. The proof and message are both encoded using the XOR function, which ensures privacy.
Zero-knowledge proofs are an essential tool in cryptography and other areas where privacy is crucial. The Zclz library makes it easy to implement zero-knowledge proofs in Python, providing a clear and concise API. By understanding and implementing zero-knowledge proofs, developers can create secure and private systems that protect sensitive data.